Introduction
Managing cloud infrastructure can be complex and time-consuming. Terraform, an open-source Infrastructure as Code (IaC) tool, simplifies this process by allowing you to define, provision, and manage your infrastructure in a declarative way. In this blog, we will explore how to use Terraform functions to automate the creation of an AWS VPC, public subnets, and EC2 instances. By leveraging Terraform functions, we can dynamically generate and manage resources efficiently.
Prerequisites
Before we dive into the code, ensure you have the following prerequisites in place:
AWS Account: You need an AWS account to create and manage resources.
Terraform Installed: Install Terraform on your local machine. You can download it from Terraform’s official site.
AWS CLI Configured: Configure the AWS CLI with your AWS credentials to allow Terraform to communicate with AWS.
Diagrammatic Representation
Understanding the Terraform Code
Let’s break down the provided Terraform code to understand how we automate AWS infrastructure.
1. Data Source for AWS Account ID
data "aws_caller_identity" "current" {}
This data source fetches the current AWS account ID. It’s useful for tagging and managing resources based on the account details.
2. Data Source for Latest Amazon Linux 2 AMI
data "aws_ami" "latest_amazon_linux" {
most_recent = true
filter {
name = "name"
values = ["amzn2-ami-hvm-*-x86_64-gp2"]
}
owners = ["amazon"]
}
This data source retrieves the latest Amazon Linux 2 AMI, ensuring that we use the most up-to-date image for our EC2 instances.
3. Data Source for Available AWS Availability Zones
data "aws_availability_zones" "available" {
state = "available"
}
This data source fetches a list of available AWS availability zones, which helps in distributing resources across multiple zones for high availability.
4. Creating a VPC
resource "aws_vpc" "customvpc" {
cidr_block = "10.0.0.0/16"
instance_tenancy = "default"
tags = {
Name = "main"
}
}
This resource block creates a Virtual Private Cloud (VPC) with a CIDR block ofĀ 10.0.0.0/16
. The VPC provides isolated networking in the AWS cloud.
5. Creating Public Subnets
resource "aws_subnet" "customsub" {
count = length(data.aws_availability_zones.available.names)
vpc_id = aws_vpc.customvpc.id
cidr_block = "10.0.${count.index}.0/24"
availability_zone = element(data.aws_availability_zones.available.names, count.index)
map_public_ip_on_launch = true
tags = {
Name = "public-${element(data.aws_availability_zones.available.names, count.index)}"
Type = "Public"
}
}
This resource block creates public subnets in each availability zone. By usingĀ count
Ā andĀ element
Ā functions, we dynamically create subnets and assign them to different availability zones, ensuring high availability and fault tolerance.
6. Creating EC2 Instances
resource "aws_instance" "custom_instances" {
count = length(aws_subnet.customsub)
ami = data.aws_ami.latest_amazon_linux.id
instance_type = "t2.micro"
subnet_id = element(aws_subnet.customsub[*].id, count.index)
tags = {
Name = "ServerNo-${count.index}"
Env = "Dev"
}
}
This resource block creates EC2 instances in the public subnets. TheĀ count
Ā andĀ element
Ā functions ensure that instances are evenly distributed across the subnets. Each instance is tagged for easy identification and management.
šāšØšāšØ YouTube Tutorial š½
Ā š„š„ Conclusion š„š„
By using Terraform functions, we can automate the creation and management of AWS resources efficiently. TheĀ count
,Ā element
, and data sources allow us to dynamically generate and distribute resources, ensuring scalability and high availability. This approach not only saves time but also reduces the risk of manual errors.
Terraform’s powerful capabilities make it an essential tool for modern cloud infrastructure management. By following this guide, you can start automating your AWS infrastructure and leverage the full potential of Terraform.
Happy automating!
š¢Ā Stay tuned for my next blog…..
So, did you find my content helpful? If you did or like my other content, feel free to buy me a coffee. Thanks.
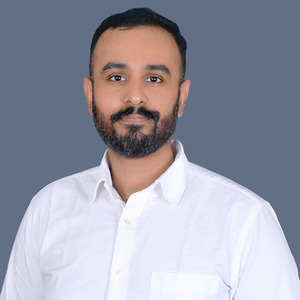
Author - Dheeraj Choudhary
RELATED ARTICLES
Automate S3 Data ETL Pipelines With AWS Glue Using Terraform
Discover how to automate your S3 data ETL pipelines using AWS Glue and Terraform in this step-by-step tutorial. Learn to efficiently manage and process your data, leveraging the power of AWS Glue for seamless data transformation. Follow along as we demonstrate how to set up Terraform scripts, configure AWS Glue, and automate data workflows.
Automating AWS Infrastructure with Terraform Functions
IntroductionManaging cloud infrastructure can be complex and time-consuming. Terraform, an open-source Infrastructure as Code (IaC) tool, si ...
Deploying a Serverless Python Flask App on AWS ECS Fargate Using Terraform
Learn how to deploy a serverless Python Flask app on AWS ECS Fargate using Terraform. This step-by-step guide covers everything from setting up your AWS environment to writing Terraform scripts and managing your Flask application.